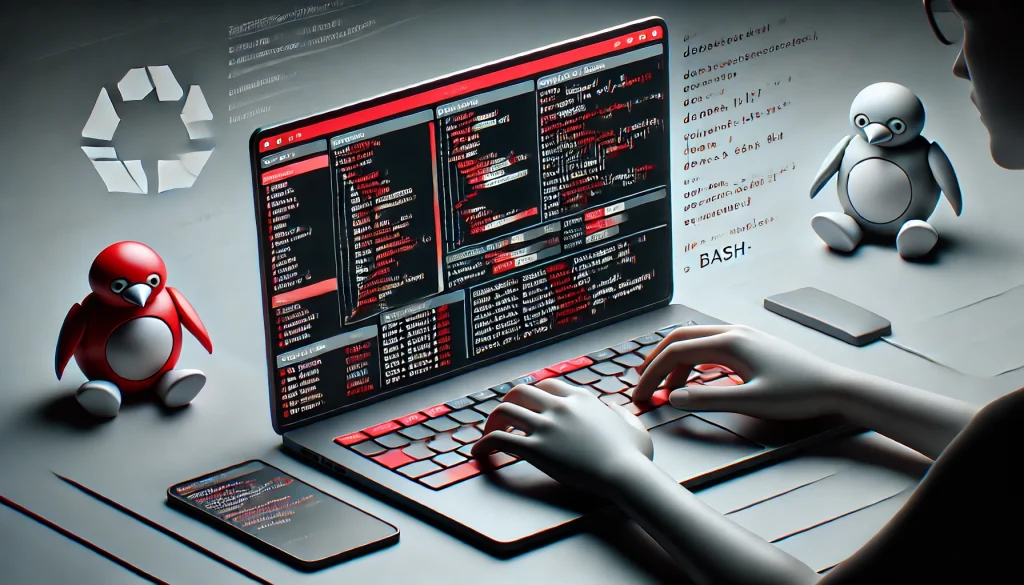
Bash scripting, a cornerstone in Linux programming, enables users to automate repetitive tasks, boost productivity, and streamline workflows. Automating workflows with Bash scripting is not just a valuable tool for developers but a powerful resource for industry professionals and enthusiasts alike. By writing scripts in the Bash shell, users can execute complex commands, manage data, and run systems with precision and ease. This guide will walk you through setting up, creating, and utilizing Bash scripts to automate tasks effectively in a Linux environment.
Materials or Tools Needed
To begin automating workflows with Bash scripts, you’ll need:
- A Linux operating system with a Bash shell (common in Ubuntu, Fedora, and Debian).
- Basic command-line knowledge.
- A text editor (such as
nano
,vim
, orgedit
). - Terminal access (either on the machine or via SSH for remote servers).
Step-by-Step Guide to Automating Workflows with Bash Scripting
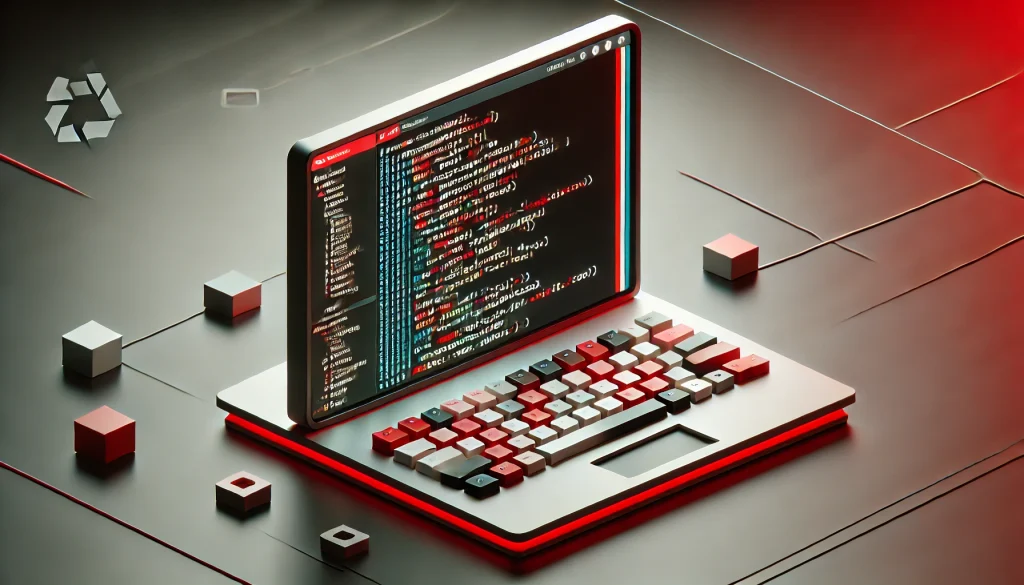
Step 1: Set Up the Script Environment
To start, open a terminal in your Linux environment and create a new file for your script. Use the command:
bashCopy codenano automate_script.sh
This command opens the nano
editor with a new file. Begin the script with the shebang #!/bin/bash
, which tells the system it’s a Bash script. Save the script using CTRL + O
, then exit with CTRL + X
.
Step 2: Define Variables and Parameters
Variables are essential in Bash scripting as they store data and make your script dynamic. Assign values using VAR_NAME="value"
and reference it later using $VAR_NAME
. For example:
bashCopy codeFILE_PATH="/path/to/directory"
echo "Path set to $FILE_PATH"
This setup allows you to define commonly used values and quickly adjust them, saving time during execution.
Step 3: Use Conditional Statements for Logic Control
Bash scripts support if-else
statements to automate decision-making. Here’s an example of a conditional statement to check if a file exists:
bashCopy codeif [ -f "$FILE_PATH" ]; then
echo "File exists."
else
echo "File not found."
fi
Conditional statements let you automate responses, making the script responsive to different inputs.
Step 4: Loop Commands for Repetitive Tasks
Loops allow you to repeat tasks. For instance, the for
loop runs a command sequence for each item in a list:
bashCopy codefor file in /path/to/files/*; do
echo "Processing $file"
done
This for
loop can be used to batch-process multiple files or execute commands across a directory.
Step 5: Automate Tasks with Cron Jobs
To make your script run automatically, schedule it using cron
. Edit the crontab
file by entering:
bashCopy codecrontab -e
Add a new line to schedule your script. For example, to run it daily at 5 a.m., add:
bashCopy code0 5 * * * /path/to/automate_script.sh
Cron jobs turn your script into a regular automated process, increasing efficiency and reducing manual intervention.
Do’s and Don’ts for Bash Scripting Automation
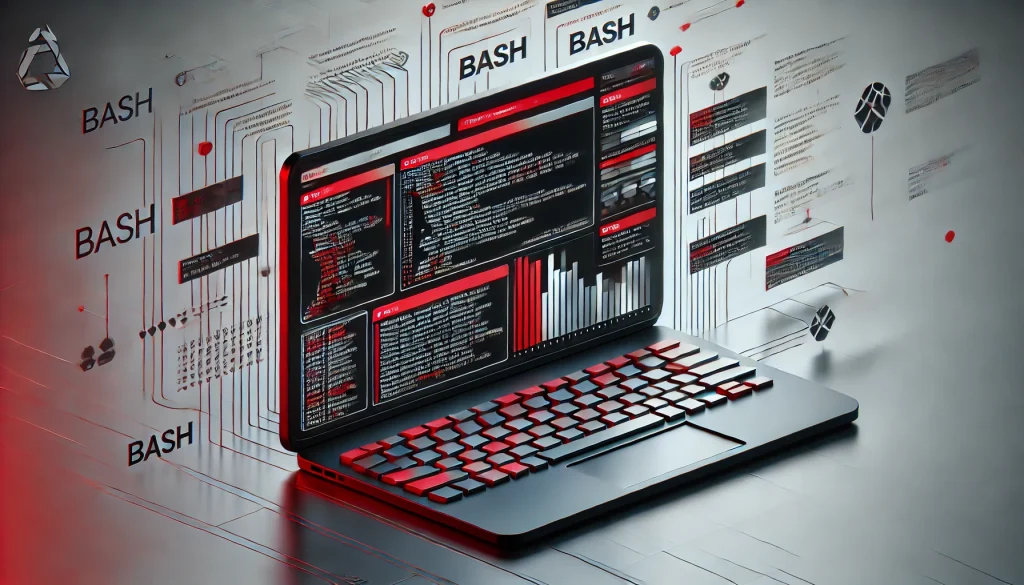
Do’s:
- Keep Scripts Simple and Modular: Break down complex workflows into smaller, manageable functions within your Bash script to enhance readability and reusability.
- Use Comments for Clarity: Comment on each function, especially if it performs multiple steps or is part of a complex sequence. Use
#
to add comments, like this:bashCopy code# This function checks for user directory
- Validate User Input: Always verify that input parameters are valid to avoid script failures or unintended behavior. Use conditional statements and error handling to ensure the script behaves as expected.
- Test Script with Sample Data: Run your script with test data to catch any errors before deploying it in a live environment.
Don’ts:
- Avoid Hardcoding Paths or Data: Instead of hardcoding paths and sensitive data within scripts, consider using environment variables or external files to keep the script flexible.
- Don’t Ignore Permissions: Make sure the script file is executable by setting the proper permissions using:bashCopy code
chmod +x automate_script.sh
- Don’t Overload the System with Unoptimized Loops: Overuse of nested loops or complex commands can slow down the script and impact system performance. Test performance with small iterations first.
Conclusion
Automating workflows with Bash scripting in Linux offers tremendous efficiency, transforming repetitive tasks into seamless operations. By setting up a script environment, leveraging conditional logic, and scheduling tasks with cron jobs, you’re on your way to mastering workflow automation in Linux. Start simple, experiment, and soon, Bash scripting will become an invaluable tool in your programming toolkit.
FAQ
What is Bash scripting in Linux?
Bash scripting is a way to automate tasks in Linux by writing and running a series of commands in the Bash shell.
Can I use Bash scripts to automate all tasks in Linux?
Not all tasks can or should be automated with Bash; it’s best suited for system tasks, data management, and other repetitive operations.
How can I debug a Bash script?
Use bash -x script.sh
to run the script in debug mode, displaying each command as it executes for easy troubleshooting.
Resources
- DraculaServers. Automate Workflows on a Linux Server with Bash Scripting.
- FreeCodeCamp. A Complete Bash Scripting Tutorial.
- LinuxHandbook. Mastering Bash Scripting for Workflow Automation.
- Medium. Getting Started with Bash Scripting: Productivity Guide.
- Noobslab. Introduction to Bash Scripting for Beginners.
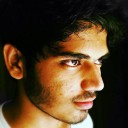
Brijesh Gohil is the founder of Tech Brij, A popular Tech Blog which is focused on Tech tips & Buying Guides. You can follow him on Facebook, Twitter, Google + & LinkedIn.